Hello tech enthusiasts! Welcome back to jasta, your go-to destination for all things tech. Today, we’re diving into how to create and use Java Objects, providing a step-by-step guide to help you accomplish this on your computer. Whether you’re a seasoned tech guru or just starting your digital journey, our straightforward instructions will make the process a breeze. Let’s jump in and start coding with Java Objects.
Table of Contents
What are Java Objects and Why Do They Exist?
As you might already know, there are some predefined objects in Java. Examples would be a String which stores some text or an Integer which can store a number. These are already pretty useful but what would you do, if you want to store multiple pieces of information for one thing? An example would be a car. All necessary information cannot be stored inside a String or an Integer. So the best approach is to create a new Object which cannot just store one particular information but many different things.
Regarding the car example some things that should be stored would be:
- Brand
- Model
- Plate
- Color
- Seats
- Steering wheel
Of course, there are many more things, but this is enough for the beginning. Some of the info can be easily stored in a string like the brand or the model whereas others require more details like the seats and steering wheel. This is because seats can come in various configurations for example with heating or without. That is also the case for the steering wheel. Therefore we would also need to create custom Java objects for the seats and the steering wheel.
Understanding the Purpose of Constructors in Java
Before actually creating your first Java Objects you have to know about constructors. A constructor is called at the very beginning of creating an object. It is called once and then never again. The purpose of it is to set some variables in the object or execute functions that are necessary at the beginning. Variables that are given with the constructor to the Java Object are often:
- Values that must be set before any method call
- Values that are already known upon the creation of the object
- Values that are used for a method call inside the constructor
To get back to the car example a constructor would look like this:
public Car(String brand, String model) { this.brand = brand; this.model = model; }
With that constructor, we are already setting the brand and the model inside the car object since this information is already known on creation. Other information is probably not known when starting with the creation of the car.
Good to know is, if there is no constructor defined in a class it is the same as an empty constructor. But there can also be multiple constructor definitions.
public Car(String brand, String model) { this.brand = brand; this.model = model; } public Car(String brand, String model, String color) { this.brand = brand; this.model = model; this.color = color; }
In the example above you have two constructors so you can create an object with these options depending on if you already know the color. In the code a new car object can now be created via ‘new Car(“Audi”, “A4”);’ or ‘new Car(“Audi”, “A4”, “Green”);’.
The difference between a constructor and a setter method is, that a constructor is once called when creating an object. A setter method can be but must not be called after initializing the object and can also be called multiple times with different values.
How to Create and Use Java Objects
Now let’s implement the car example with Java Objects. The complete code can be found and cloned from the jasta GitHub repository. To begin with we have to create our objects by creating new classes. If you don’t know how to create classes in IntelliJ IDEA click here. We need to create the following classes: Car, Seat, Steeringwheel and a Main class to test the Java Objects. After creating all classes your project structure looks like the screenshot below.
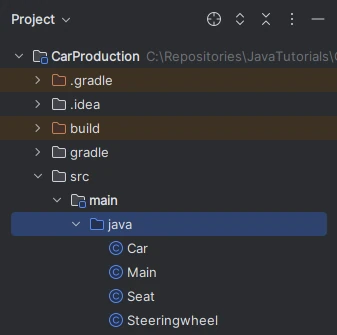
First of all, we are adding code to the seat class. We are adding three variables to the class: material, weight and heating. Since we will probably know all of these when creating the seat we are setting all of them inside the constructor. Also, these attributes will not change, therefore they have the final keyword in front of the declaration so they can not be modified later on. To get the values of the variables we are also adding get methods.
public class Seat { private final String material; private final int weight; private final boolean heating; public Seat(String material, int weight, boolean heating) { this.material = material; this.weight = weight; this.heating = heating; } public String getMaterial() { return material; } public int getWeight() { return weight; } public boolean isHeating() { return heating; } }
The second class which will be implemented is the Steeringwheel class. For simplicity, we are just adding two variables: material and diameter. These will be known on creation and should not be changed later. Therefore the final keyword is added again and the values are assigned inside the constructor. In addition, of course, getter methods are added.
public class Steeringwheel { private final String material; private final int diameter; public Steeringwheel(String material, int diameter) { this.material = material; this.diameter = diameter; } public String getMaterial() { return material; } public int getDiameter() { return diameter; } }
Now that all Java Objects used for the car object are created, we can implement the car class. As previously described a car will have multiple seats therefore a list of seats, a String brand, a String model, a Steeringwheel object, a String plate and a String with the car’s color. The list type, the brand and the model shouldn’t be modified after initialization therefore they are declared final. Since the list type is already declared at the variable declaration only the brand and the model need to be set in the constructor. Every other attribute can be set via the corresponding set method. Of course getter methods are added as well.
import java.util.ArrayList; import java.util.List; public class Car { private final List<Seat> seats = new ArrayList<>(); private final String brand; private final String model; private Steeringwheel steeringwheel; private String plate; private String color; public Car(String brand, String model) { this.brand = brand; this.model = model; } public List<Seat> getSeats() { return seats; } public void addSeat(Seat seat) { seats.add(seat); } public String getBrand() { return brand; } public String getModel() { return model; } public Steeringwheel getSteeringwheel() { return steeringwheel; } public void setSteeringwheel(Steeringwheel steeringwheel) { this.steeringwheel = steeringwheel; } public String getPlate() { return plate; } public void setPlate(String plate) { this.plate = plate; } public String getColor() { return color; } public void setColor(String color) { this.color = color; } }
To test and understand how all this works, you can copy the code from below into your main class and execute it.
public class Main { public static void main(String[] args) { Car audiA4 = new Car("Audi", "A4"); audiA4.setColor("green"); Steeringwheel steeringwheel = new Steeringwheel("Leather", 38); audiA4.setSteeringwheel(steeringwheel); audiA4.addSeat(new Seat("Leather", 10, true)); audiA4.addSeat(new Seat("Leather", 10, true)); audiA4.addSeat(new Seat("Fabric", 8, false)); audiA4.addSeat(new Seat("Fabric", 8, false)); audiA4.addSeat(new Seat("Fabric", 8, false)); audiA4.setPlate("ABCD1"); System.out.println( "Car: " + audiA4.getBrand() + " " + audiA4.getModel() + " in " + audiA4.getColor()); System.out.println( "Steeringwheel: " + audiA4.getSteeringwheel().getMaterial() + " steeringwheel which is " + audiA4.getSteeringwheel().getDiameter() + "cm big"); int count = 1; String tempSeatOutput; for (Seat seat : audiA4.getSeats()) { tempSeatOutput = "Seat " + count + ": " + seat.getMaterial() + " seat which weights " + seat.getWeight() + "kg"; if (seat.isHeating()) { tempSeatOutput += " and can heat up"; } else { tempSeatOutput += " and cannot heat up"; } System.out.println(tempSeatOutput); count++; } System.out.println("Plate: " + audiA4.getPlate()); } }
The code above creates a new object with Car audiA4 = new Car(“Audi”, “A4”);. ‘Audi’ and ‘A4’ are the constructor arguments (brand and model). After creation, we are now using the setter methods of the car objects to set other variables.
The color is just set with a simple String argument. To set a Steeringwheel object we first have to create one. This is done like the Car object but now with Steeringwheel: Steeringwheel steeringwheel = new Steeringwheel(“Leather”, 38);. When creating the Steeringwheel Object the constructor arguments (material and diameter) are added. Now there are two separate objects. One is the Car and the other one is the Steeringwheel Object. To link the Steeringwheel object to the Car object we need to call the setSteeringwheel method of the car. The same process is now done with the seats but with anonymous objects.
Anonymous objects are used if you don’t need to access the object later on in the code. So you can type new Seat(<material>, <weight>, <heating>) and add it immediately to the car with addSeat. This has two benefits. All this is done within one line and you don’t have to think about an object name. This can also be done with the Steeringwheel object since it will not be used directly later in the code.
After setting the plate every information about the car is printed out in the console. To do this the respective getter method is called to get the wanted String, Steeringwheel or Seat objects.
That’s the process of creating and using Java Objects. If you have any questions feel free to leave a comment.